Mastering CSS animations can transform static websites into dynamic, engaging experiences. This guide delves into seven creative ideas, showcasing both fundamental and advanced techniques. From simple fade-ins to complex parallax scrolling, we’ll explore how to leverage CSS to add captivating motion to your web designs, enhancing user interaction and overall aesthetic appeal.
We’ll cover essential concepts like `@keyframes`, timing functions, and CSS transforms, providing clear code examples and explanations throughout. We’ll also compare CSS animations to JavaScript alternatives, discussing performance implications to help you make informed decisions for your projects. Whether you’re a beginner or seeking to expand your animation skills, this guide offers a practical and insightful journey into the world of CSS-powered web motion.
Fundamental CSS Animation Techniques
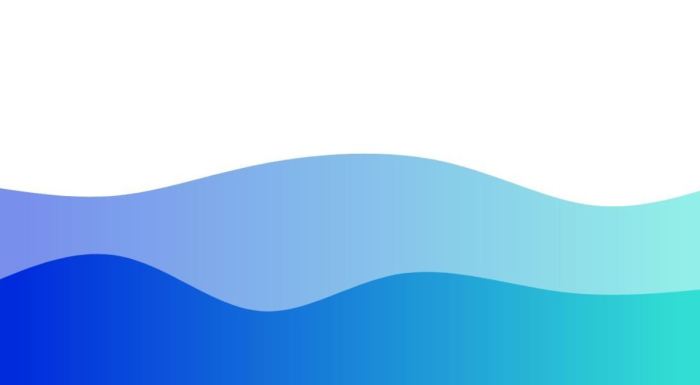
CSS animations offer a powerful way to enhance user engagement and create visually appealing web experiences. By leveraging CSS properties and the `@keyframes` rule, developers can craft dynamic and interactive elements without relying on JavaScript. This section explores core techniques for creating effective CSS animations.
Fade-in Effect on an Image
A simple fade-in effect is a common animation used to smoothly introduce an image onto the page. This can be achieved using the `opacity` property within `@keyframes`. Different timing functions, such as `ease`, `ease-in-out`, and `linear`, control the speed and pacing of the animation.
/* CSS */
.fade-in-image
animation: fadeIn 1s ease; /* 1 second duration, ease timing function */
@keyframes fadeIn
from opacity: 0;
to opacity: 1;
/* HTML */
Replacing `ease` with `ease-in-out` creates a smoother start and end, while `linear` results in a constant animation speed. Experimenting with different timing functions allows for fine-tuning the visual impact.
Bouncing Ball Effect
Animating a bouncing ball requires controlling both vertical position and potentially scale or even visual properties like a shadow effect to enhance the realism of the animation. Keyframes define the different states of the animation, and the `animation` property sets the animation’s name, duration, and timing function.
/* CSS */
.bouncing-ball
animation: bounce 2s infinite ease-in-out; /* 2 second duration, infinite iterations */
width: 50px;
height: 50px;
background-color: blue;
border-radius: 50%;
@keyframes bounce
0% transform: translateY(0);
50% transform: translateY(-100px) scale(1.2); /* Scale for visual emphasis */
100% transform: translateY(0);
/* HTML */
The `transform: translateY()` property controls the vertical position of the ball, while `scale()` adds a slight enlargement at the peak of the bounce. The `infinite` iteration count ensures continuous bouncing.
Controlling Animation Sequences with `@keyframes`
The `@keyframes` rule is central to CSS animations. It defines the animation’s progression through a series of keyframes, each specified by a percentage (0%, 25%, 50%, 75%, 100%) or specific time (e.g., 1s, 2s). Different iteration counts (`infinite`, numerical values) control how many times the animation repeats. `infinite` causes the animation to loop continuously, while a numerical value specifies a finite number of repetitions.
For example, setting `animation-iteration-count: 3;` will play the animation three times. The choice depends on the desired effect; infinite loops are suitable for loading indicators or background animations, while finite loops are better for short, self-contained animations.
Simple Loading Animation
A simple loading animation can be created using a rotating element. This example uses a `div` with a border radius to simulate a circular spinner.
CSS | HTML |
---|---|
|
|
This code creates a rotating circle. The `border-top` property is used to create a colored section, which appears to rotate due to the `transform: rotate()` property within the `@keyframes` rule. The `linear` timing function ensures a consistent rotation speed.
Advanced CSS Animation Concepts and Practical Applications
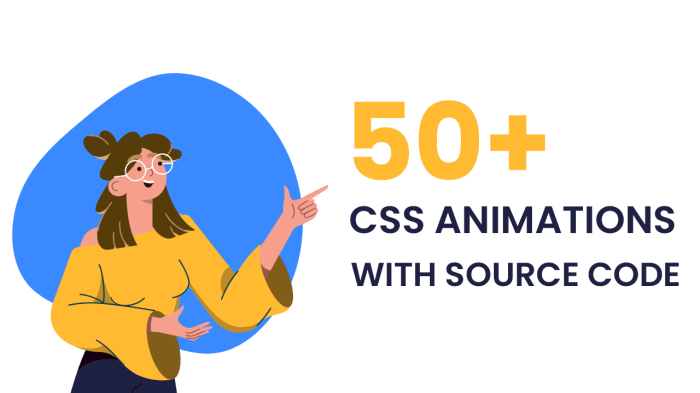
CSS animations offer a powerful way to enhance user experience and create engaging web designs. Moving beyond basic animations, understanding advanced techniques unlocks a wider range of possibilities for creating dynamic and visually appealing interfaces. This section explores key concepts and their practical applications.
CSS Transforms in Animations
CSS transforms—`scale`, `rotate`, and `translate`—provide efficient ways to manipulate an element’s position and appearance without affecting the document layout. Combining these transforms within animations creates complex and visually interesting effects. For instance, you can animate an element to scale up while simultaneously rotating and translating it across the screen. This creates a more dynamic and engaging animation than simply scaling or rotating alone.
Consider the following example:
.animated-element
animation: myAnimation 2s ease-in-out;
@keyframes myAnimation
0% transform: scale(0.5) rotate(0deg) translateX(0);
50% transform: scale(1.2) rotate(360deg) translateX(100px);
100% transform: scale(1) rotate(720deg) translateX(200px);
This code animates an element over two seconds, smoothly scaling it up and down, rotating it twice, and moving it across the screen. The `ease-in-out` timing function ensures a smooth, natural-looking transition.
Parallax Scrolling with CSS Animations
Parallax scrolling creates a sense of depth by making background elements move at a slower rate than foreground elements as the user scrolls. This effect enhances visual interest and can add a professional touch to websites. While often implemented with JavaScript, CSS animations can achieve a simplified parallax effect, particularly for less complex scenarios. This involves animating the `transform: translateX` or `transform: translateY` property of background elements based on the scroll position.
To achieve this, you’ll need to use the `scroll` event and JavaScript to get the scroll position. Then, you’ll update the `transform` property of your background elements using JavaScript. While purely CSS-based parallax is limited, this method allows for a smooth and efficient parallax effect within the limitations of CSS.
Performance Comparison: CSS Animations vs. JavaScript Animations
Both CSS animations and JavaScript animations can create dynamic visual effects, but they differ significantly in performance. CSS animations are generally more performant as they are handled directly by the browser’s rendering engine, resulting in smoother animations and less strain on the CPU. JavaScript animations, on the other hand, require more processing power because they manipulate the DOM directly, potentially leading to performance bottlenecks, especially with complex animations or a large number of elements.
For simple animations involving a small number of elements, the performance difference might be negligible. However, for complex animations or when animating many elements, CSS animations will generally offer better performance. Choosing the right approach depends on the complexity of the animation and the overall performance requirements of the website.
Animated Navigation Menu
A responsive navigation menu with animated transitions on hover provides a visually engaging and user-friendly experience. The following example utilizes a 3-column table to structure the menu, demonstrating CSS for styling and hover animations.
Menu Item | CSS Styles | Hover Animation |
---|---|---|
Home | background-color: #f0f0f0; padding: 10px; border: 1px solid #ccc; |
transition: background-color 0.3s ease; background-color: #ddd; transform: scale(1.1); |
About | background-color: #f0f0f0; padding: 10px; border: 1px solid #ccc; |
transition: background-color 0.3s ease; background-color: #ddd; transform: scale(1.1); |
Contact | background-color: #f0f0f0; padding: 10px; border: 1px solid #ccc; |
transition: background-color 0.3s ease; background-color: #ddd; transform: scale(1.1); |
This table showcases a simple navigation menu with a subtle hover effect. The `transition` property smoothly animates the background color change and scaling on hover, providing a smooth user experience. More complex animations can be achieved by using `@keyframes` for more intricate transitions.
Creative CSS Animation Ideas for Web Design
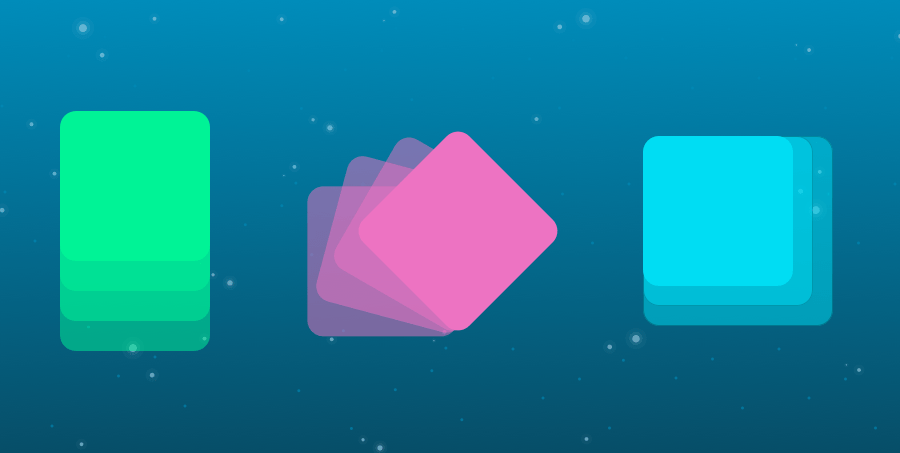
CSS animations offer a powerful way to enhance user experience and create engaging web designs. By thoughtfully incorporating motion, we can elevate otherwise static elements, making interactions more intuitive and visually appealing. This section explores several creative applications of CSS animations, providing code examples and explanations to guide you in implementing these techniques.
Shape-Shifting Button
This example demonstrates a button that changes its shape and color on hover. The animation is achieved using CSS transitions and transforms. The key is to define different states for the button (default and hover) and use transitions to smoothly animate between them.
/* Default button style */
.shape-shifting-button
background-color: #4CAF50;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
border-radius: 5px;
transition: all 0.3s ease; /* Smooth transition for all properties */
/* Hover state style */
.shape-shifting-button:hover
background-color: #3e8e41;
border-radius: 20px; /* Change shape on hover */
transform: scale(1.1); /*Slight enlargement*/
This code uses a `transition` property to smoothly animate changes in background color and border radius over 0.3 seconds. The `transform: scale(1.1)` subtly enlarges the button on hover, adding a further dynamic effect.
Animated Progress Bar
A progress bar visually communicates the completion status of a task. Using CSS animations, we can create a dynamic and engaging progress bar. CSS variables allow for easy customization of the animation speed and maximum progress value.
.progress-bar
width: 100%;
background-color: #f4f4f4;
border-radius: 5px;
overflow: hidden; /* Hide overflowing fill */
.progress-bar-fill
height: 20px;
background-color: #4CAF50;
width: 0%; /* Initially empty */
animation: progress 5s linear forwards; /* Animate over 5 seconds */
/* CSS variable for dynamic control */
:root
--progress-max: 100%;
@keyframes progress
to
width: var(--progress-max); /* Fill to the maximum value */
The `–progress-max` variable allows us to easily adjust the final width of the progress bar, offering flexibility in its use. The `linear` ensures a consistent animation speed.
Engaging Loading Screen
A visually appealing loading screen can greatly improve the user experience by providing a sense of progress and preventing a blank screen. CSS animations can create captivating loading animations, for example, a rotating spinner or a pulsating circle.
.loading-screen
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5); /* Semi-transparent overlay */
display: flex;
justify-content: center;
align-items: center;
.loading-spinner
width: 50px;
height: 50px;
border: 5px solid #f3f3f3; /* Light gray border */
border-top: 5px solid #3498db; /* Blue top border */
border-radius: 50%;
animation: spin 1s linear infinite; /* Rotate continuously */
@keyframes spin
0% transform: rotate(0deg);
100% transform: rotate(360deg);
This code creates a simple, rotating spinner. The `rgba` function is used to make the background semi-transparent, allowing the underlying content to be partially visible.
Subtle Micro-interaction: Form Submission
Micro-interactions provide subtle feedback to user actions, enhancing the overall experience. A smooth transition on form submission is a perfect example.
HTML | CSS | JavaScript (Optional) | Description |
---|---|---|---|
|
|
|
On click, the button adds the ‘submitted’ class, triggering a scale and opacity change. JavaScript handles the actual form submission. |
This example uses a class toggle to control the animation. The CSS transitions provide a smooth scaling and fading effect. JavaScript could be used to remove the class after a delay or successful submission.
Closing Summary
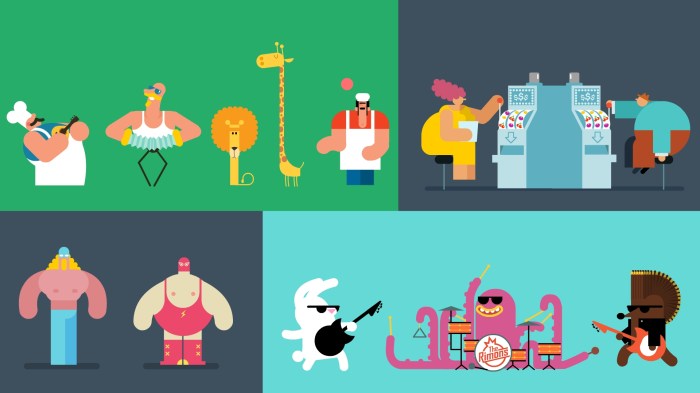
By exploring these seven diverse CSS animation techniques, you’ve gained a powerful toolkit for enriching your web designs. Remember, the key is to use animation purposefully – enhancing user experience, not distracting from it. Experiment with different timing functions, transforms, and keyframes to create unique and engaging motion effects that elevate your projects. The possibilities are vast, so start animating!